General questions
| |
TheCheeseLover | Date: Saturday, 2009-05-16, 2:14 AM | Message # 1 |
 Sergeant
Group: Users
Messages: 22
Status: Offline
| Hi! I posted this for every one who want to ask a question and who don't want to start a new thread. I will start 1: Is there any different actions then ''pick every unit in area'' without the leak?
Yay I have a signature. You suck. :D
|
|
| |
Fireeye | Date: Sunday, 2009-05-17, 2:35 PM | Message # 2 |
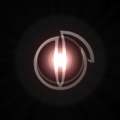 Private
Group: Moderators
Messages: 16
Status: Offline
| 1: Only when you use the JASS / vJASS way. You basically use a global variable, GroupEnumUnitsXXX and GroupClear. Let me post some pseudo code for explanation. Code globals group tmp_group = CreateGroup() endglobals
function ClearGroupAlternate takes nothing returns nothing //Whatever call GroupRemoveUnit(tmp_group,GetEnumUnit()) endfunction
function ClearGroupAlternateCall takes nothing returns nothing call GroupEnumUnitsOfPlayer(tmp_group,Player(0),null) //Note that 'null' will cause a "leak" (most likely a fake one) if used as boolexpr call ForGroup(tmp_group,function ClearGroupAlternate) endfunction
function ClearGroup takes nothing returns nothing //Whatever endfunction
function ClearGroupCall takes nothing returns nothing call GroupEnumUnitsOfPlayer(tmp_group,Player(0),null) //Note that 'null' will cause a "leak" (most likely a fake one) if used as boolexpr call ForGroup(tmp_group,function ClearGroup) call GroupClear(tmp_group) endfunction I posted 2 different ways of removing units from a unit group. When we use the JASS functions for enuming units, there are no CreateGroup() calls, therefore we can use the same handle id => same group over and over (also called recycling). If you want to see the GUI functions, here they are, well only some of them, but you'll see why GUI sucks. Code function GetUnitsInRangeOfLocAll takes real radius, location whichLocation returns group return GetUnitsInRangeOfLocMatching(radius, whichLocation, null) endfunction function GetUnitsInRangeOfLocMatching takes real radius, location whichLocation, boolexpr filter returns group local group g = CreateGroup() call GroupEnumUnitsInRangeOfLoc(g, whichLocation, radius, filter) call DestroyBoolExpr(filter) return g endfunction function GetUnitsInRectAll takes rect r returns group return GetUnitsInRectMatching(r, null) endfunction function GetUnitsInRectMatching takes rect r, boolexpr filter returns group local group g = CreateGroup() call GroupEnumUnitsInRect(g, r, filter) call DestroyBoolExpr(filter) return g endfunction function GetUnitsInRectOfPlayer takes rect r, player whichPlayer returns group local group g = CreateGroup() set bj_groupEnumOwningPlayer = whichPlayer call GroupEnumUnitsInRect(g, r, filterGetUnitsInRectOfPlayer) return g endfunction So to eliminate the leak you have to stop using the GUI equivalent and use the JASS one. Code native GroupEnumUnitsInRange takes group whichGroup, real x, real y, real radius, boolexpr filter returns nothing native GroupEnumUnitsInRangeCounted takes group whichGroup, real x, real y, real radius, boolexpr filter, integer countLimit returns nothing native GroupEnumUnitsInRangeOfLoc takes group whichGroup, location whichLocation, real radius, boolexpr filter returns nothing native GroupEnumUnitsInRangeOfLocCounted takes group whichGroup, location whichLocation, real radius, boolexpr filter, integer countLimit returns nothing native GroupEnumUnitsInRect takes group whichGroup, rect r, boolexpr filter returns nothing native GroupEnumUnitsInRectCounted takes group whichGroup, rect r, boolexpr filter, integer countLimit returns nothing native GroupEnumUnitsOfPlayer takes group whichGroup, player whichPlayer, boolexpr filter returns nothing native GroupEnumUnitsOfType takes group whichGroup, string unitname, boolexpr filter returns nothing native GroupEnumUnitsOfTypeCounted takes group whichGroup, string unitname, boolexpr filter, integer countLimit returns nothing native GroupEnumUnitsSelected takes group whichGroup, player whichPlayer, boolexpr filter returns nothing
Message edited by Fireeye - Sunday, 2009-05-17, 2:35 PM |
|
| |
TheCheeseLover | Date: Sunday, 2009-07-19, 3:02 PM | Message # 3 |
 Sergeant
Group: Users
Messages: 22
Status: Offline
| Hi! Code library Thecheeselover //Function: //function ScriptFallSheep takes real howmuch, real timeout, unit wichu returns nothing //function ScriptSlide takes unit wichu, location point, real duration, real z, boolean bool returns nothing //function ScriptMakeUnitFly takes unit wichunit returns nothing // Global functions function ScriptMakeUnitFly takes unit wichunit returns nothing call UnitAddAbility(wichunit, 'Amrf') call UnitRemoveAbility(wichunit, 'Amrf') endfunction //End global functions //start sheep globals private timer sheeptt = CreateTimer() private timer sheept = CreateTimer() private unit sheepu endglobals function SheepStop takes nothing returns nothing call PauseTimer(sheept) call DestroyTimer(sheept) call DestroyTimer(sheeptt) set sheepu = null endfunction
function sheep takes nothing returns nothing local effect sheepp local location p = GetRandomLocInRect(GetPlayableMapRect()) call AddSpecialEffectLocBJ( p, "Abilities\\Spells\\Human\\Polymorph\\PolyMorphFallingSheepArt.mdl" ) set sheepp = GetLastCreatedEffectBJ() call UnitDamagePoint(sheepu, 1, 80, GetLocationX(p), GetLocationY(p), 100, TRUE, FALSE, ATTACK_TYPE_MAGIC, DAMAGE_TYPE_UNKNOWN, WEAPON_TYPE_WOOD_LIGHT_BASH) call DestroyEffect(sheepp) call RemoveLocation(p) endfunction
function ScriptFallSheep takes real howmuch, real timeout, unit wichu returns nothing local real sec = 1.00 local real number = howmuch/sec set sheepu = wichu call TimerStart(sheept, number, TRUE, function sheep) call TimerStart(sheeptt, timeout, FALSE, function SheepStop) endfunction //End sheep // Start slide globals private unit SlideUnit = null private real SlidePointX = 0.00 private real SlidePointY = 0.00 private real SlidePointZ = 0.00 private real SlideGuyX = 0.00 private real SlideGuyY = 0.00 private real SlideDuration = 0.00 private real SlideX = 0.00 private real SlideY = 0.00 private timer SlideT = CreateTimer() private real SlideSecond = 0.00 private real SlideR = 0.00 private boolean SlideBool = FALSE private real USpeed = 0.00 endglobals function SlideStop takes nothing returns nothing call ScriptMakeUnitFly(SlideUnit) call PauseUnit(SlideUnit, FALSE) call SetUnitFlyHeight(SlideUnit, 0, 1000000) set SlideUnit = null set SlidePointX = 0.00 set SlidePointZ = 0.00 set SlidePointY = 0.00 set SlideGuyX = 0.00 set SlideGuyY = 0.00 set SlideDuration = 0.00 set SlideX = 0.00 set SlideY = 0.00 set SlideSecond = 0.00 set SlideR = 0.00 set USpeed = 0.00 endfunction function Slide takes nothing returns nothing set SlideGuyX = GetUnitX(SlideUnit) set SlideGuyY = GetUnitY(SlideUnit) call SetUnitX(SlideUnit, SlideGuyX+SlideX) call SetUnitY(SlideUnit, SlideGuyY+SlideY) call DisplayTimedTextToForce( GetPlayersAll(), 5.00, R2S(SlideY) ) call DisplayTimedTextToForce( GetPlayersAll(), 5.00, R2S(SlideX) ) if SlideR == 0.00 then call ScriptMakeUnitFly(SlideUnit) call SetUnitFlyHeight(SlideUnit, SlidePointZ, SlidePointZ / SlideDuration / 2) else endif if SlideR == SlideDuration / 2 then call ScriptMakeUnitFly(SlideUnit) call SetUnitFlyHeight(SlideUnit, 0, SlidePointZ / SlideDuration / 2) else endif if SlideR > SlideDuration then call PauseTimer(SlideT) call SlideStop() else endif set SlideR = SlideR + 0.01 endfunction function ScriptSlide takes unit wichu, location point, real duration, real z, boolean bool returns nothing set SlideUnit = wichu set USpeed = GetUnitMoveSpeed(SlideUnit) set SlideBool = bool set SlideGuyX = GetUnitX(SlideUnit) set SlideGuyY = GetUnitY(SlideUnit) set SlidePointX = GetLocationX(point) set SlidePointY = GetLocationY(point) set SlidePointZ = z set SlideDuration = duration set SlideSecond = 0.01 //SlidePointX - SlideGuyX / SlideDuration * SlideSecond set SlideX = SlidePointX set SlideX = SlideX - SlideGuyX set SlideX = SlideX / SlideDuration set SlideX = SlideX * SlideSecond set SlideX = SlideX * 1 set SlideY = SlidePointY set SlideY = SlideY - SlideGuyY set SlideY = SlideY / SlideDuration set SlideY = SlideY * SlideSecond set SlideY = SlideY * 1 if SlideBool == TRUE then call PauseUnit(SlideUnit, TRUE) else endif call TimerStart(SlideT, 0.01, TRUE, function Slide) call RemoveLocation(point) set wichu = null set duration = 0.00 set z = 0.00 endfunction //End Slide endlibrary Here I made a library but like usually there are some problems. The function "ScriptFallSheep" doesn't work and I don't know why. Also, the function "ScriptSlide" bugs every time except the first one. The bug is that the unit doesn't get the ability Amrf. Help Added (2009-07-19, 3:02 Pm) --------------------------------------------- Nvm for my library. Now I have another question. Code library_once Peanut initializer peanutInit What does it do if I use ''library_once'' to start my library?
Yay I have a signature. You suck. :D
Message edited by TheCheeseLover - Sunday, 2009-07-19, 3:04 PM |
|
| |
Fireeye | Date: Sunday, 2009-07-19, 4:53 PM | Message # 4 |
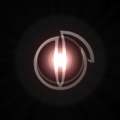 Private
Group: Moderators
Messages: 16
Status: Offline
| Reading the Jass Helper Manual can help often. Here's the answer: The library_once keyword works exactly like library but you can declare the same library name twice, it would just ignore the second declaration and avoid to add its contents instead of showing a syntax error, it is useful in combination with textmacros.
|
|
| |
TheCheeseLover | Date: Friday, 2009-07-24, 3:21 PM | Message # 5 |
 Sergeant
Group: Users
Messages: 22
Status: Offline
| Hi again! I have two more questions about units in area. What does it do ''boolexpr''? (I know that it is a boolean) How can we pick all units or a number of units in a group and do actions with them without leaking? (Usually in Gui it is ''Pick every units in group'' and then you do your actions saying that the unit is PickedUnit) PS: Now I see why Jass sucks.
Yay I have a signature. You suck. :D
Message edited by TheCheeseLover - Friday, 2009-07-24, 3:30 PM |
|
| |
Fireeye | Date: Friday, 2009-07-24, 7:55 PM | Message # 6 |
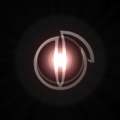 Private
Group: Moderators
Messages: 16
Status: Offline
| boolexpr does not really 'leak', they use up space in the memory, however they're kinda special case. Let's say you use Condition(function x) and the same thing somewhere else in the map, only 1 boolexpr will be created. However if you destroy 1 boolexpr and the same condition is used somewhere else in the map it'll get destroyed too. When you use it as filter, you can use GetFilterUnit() to get a unit before it's picked e.g. GroupEnumUnitsInRange(). When you got your group and use the command ForGroup(), you can get the 'picked unit# with GetEnumUnit().
|
|
| |
TheCheeseLover | Date: Saturday, 2009-07-25, 5:24 PM | Message # 7 |
 Sergeant
Group: Users
Messages: 22
Status: Offline
| Thanks. Does it do anything if I don't use boolexpr because it's really hard to understand? :/
Yay I have a signature. You suck. :D
|
|
| |
Hanky | Date: Friday, 2009-08-07, 10:40 AM | Message # 8 |
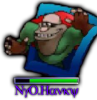 Lieutenant colonel
Group: Moderators
Messages: 116
Status: Offline
| Not really but in some situations it would be better.
Made by Smoe reworked by GiR aka Darkt3mpl3r
|
|
| |
TheCheeseLover | Date: Saturday, 2009-08-29, 4:47 AM | Message # 9 |
 Sergeant
Group: Users
Messages: 22
Status: Offline
| Oops, I saw a mistake on a post and I cant edit it. ''PS: Now I see why Jass sucks.''. I wanted to write this: ''Now I see why Gui sucks''.Added (2009-08-29, 4:47 Am) --------------------------------------------- Sorry for double postiong but I have a question. :P How do you make a system safety?
Yay I have a signature. You suck. :D
Message edited by TheCheeseLover - Wednesday, 2009-08-12, 8:24 PM |
|
| |
Darkt3mpl3r | Date: Saturday, 2009-08-29, 7:05 PM | Message # 10 |
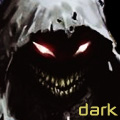 Sergeant
Group: Administrators
Messages: 35
Status: Offline
| for example by preventing to hit the max array size. build in a integer that checks if the current stack ( if you're using indexing ) is below 8191 ..
|
|
| |
Inferior | Date: Wednesday, 2009-09-09, 10:26 PM | Message # 11 |
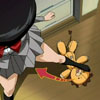 Private
Group: Checked
Status: Offline
| hey there guys. Got a problem with my map. All the time i test it for multiplayability with my brother he gets kicked off the game. I dont know if there is a fault in my script or if its just a bug. Well, i would be glad if someone of you could help me with it. ~Inferior
I'm just one of those wannabe nerds who want to create a map
|
|
| |
WaRadius | Date: Wednesday, 2009-09-09, 10:38 PM | Message # 12 |
 Admin
Group: Administrators
Messages: 27
Status: Offline
| I remember there was one server split bug with GetLocalPlayer(), but can't remember what it was exactly.
|
|
| |
Inferior | Date: Wednesday, 2009-09-09, 11:28 PM | Message # 13 |
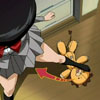 Private
Group: Checked
Status: Offline
| love ya. that could be the prob. Well seems i have to change sum script stuff.
I'm just one of those wannabe nerds who want to create a map
|
|
| |
WaRadius | Date: Thursday, 2009-09-10, 12:38 PM | Message # 14 |
 Admin
Group: Administrators
Messages: 27
Status: Offline
| bj values/functions + GetLocalPlayer() = split
|
|
| |
Inferior | Date: Thursday, 2009-09-10, 8:16 PM | Message # 15 |
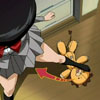 Private
Group: Checked
Status: Offline
| well i try to avoid as much bj functions as i can. and if i think of it dont use any bj functions. Will check for bj values which can be replaced thx again WaRa ~Inferior
I'm just one of those wannabe nerds who want to create a map
|
|
| |
|