[vJASS] Some systems ...
| |
Fireeye | Date: Thursday, 2008-10-30, 2:33 PM | Message # 1 |
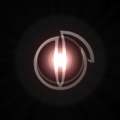 Private
Group: Moderators
Messages: 16
Status: Offline
| Well, then here are some systems i used, all written by myself, so gl & hf understanding the code. I might write a ReadMe later but not atm. only needed if you got no gamecache library Available for users only CheckLoc function Available for users only LightningFade library Code library LF initializer Init //*********************************************************************************************************************** //* UCP Beginning (User configuration Part) * //*********************************************************************************************************************** globals p rivate constant real UPDATE_TIME = .02 private constant boolean DESTROY_ON_TIME_OUT = true private constant boolean DEBUG_MODE = true endglobals //*********************************************************************************************************************** //* UCP Ending * //*********************************************************************************************************************** private keyword LightningFade globals private timer t = CreateTimer() private LightningFade array gdat private integer current_slot = 0 endglobals struct LightningFade lightning l = null boolean Destroy = false real array InitValue [4] real array TimeFactor [4] real current_time = 0. real end_time = 0. static method New takes lightning l, real time, boolean b, real r1, real g1, real b1, real a1, real r2, real g2, real b2, real a2 returns nothing local LightningFade d = LightningFade.create() if l != null then set d.l = l set d.Destroy = b set d.InitValue[0] = r1 set d.InitValue[1] = g1 set d.InitValue[2] = b1 set d.InitValue[3] = a1 set d.TimeFactor[0] = r1-r2 set d.TimeFactor[1] = g1-g2 set d.TimeFactor[2] = b1-b2 set d.TimeFactor[3] = a1-a2 set d.end_time = time set gdat[current_slot] = d set current_slot = current_slot + 1 if DEBUG_MODE then call BJDebugMsg("Current Amount of Lightnings hold: "+I2S(current_slot-1)) endif else if DEBUG_MODE then call BJDebugMsg("Lightning passed as null argument!") endif endif endmethod static method NewEX takes lightning l, real time, boolean b, real r2, real g2, real b2, real a2 returns nothing call LightningFade.New(l,time,b,GetLightningColorR(l),GetLightningColorG(l),GetLightningColorB(l),GetLightningColorA(l),r2,g2,b2,a2) e n dmethod method onDestroy takes nothing returns nothing if DESTROY_ON_TIME_OUT or .Destroy then call DestroyLightning(.l) set .l = null endif endmethod endstruct private function UpdateLightning takes nothing returns nothing local LightningFade d local integer i = 0 local real r = 0 local real g = 0 local real b = 0 local real a = 0 loop exitwhen i == current_slot set d = gdat[i] set d.current_time = d.current_time + UPDATE_TIME if d.current_time < d.end_time then set r = d.InitValue[0]+d.current_time*d.TimeFactor[0] set g = d.InitValue[1]+d.current_time*d.TimeFactor[1] set b = d.InitValue[2]+d.current_time*d.TimeFactor[2] set a = d.InitValue[3]+d.current_time*d.TimeFactor[3] if DEBUG_MODE then call BJDebugMsg("Current color values of lightning |cffff0000"+I2S(i)+"|r is : |cffff0000"+I2S(R2I(r*255))+" |cff00ff00"+I2S(R2I(g*255))+" |cff0000ff"+I2S(R2I(b*255))+" |cff00000"+I2S(R2I(a*255))+"r") endif call SetLightningColor(d.l,r,g,b,a) set i = i + 1 else call d.destroy() set current_slot = current_slot - 1 set gdat[i] = gdat[current_slot] endif endloop endfunction private function Init takes nothing returns nothing call TimerStart(t,UPDATE_TIME,true,function UpdateLightning) endfunction endlibrary Bonus Stats library (only works for heros!) Code library BonusStats initializer Init requires GameCache globals private trigger tr = null endglobals private struct Data unit hero boolean reset real array StatsTotal[3] real array StatsTemp[3] real array PartStats[3] endstruct public function Add takes unit u, integer whichstat, real value, boolean temp returns nothing local integer i = H2I(u) local string s = I2S(i) local Data dat if GetStoredBoolean(gc,s+"BonusStats","Exist") then set dat = Data(GetStoredInteger(gc,s+"BonusStats","Struct")) else call StoreBoolean(gc,s+"BonusStats","Exist",true) set dat = Data.create() call StoreInteger(gc,s+"BonusStats","Struct",integer(dat)) endif set dat.StatsTotal[whichstat] = dat.StatsTotal[whichstat] + value if temp then set dat.StatsTemp[whichstat] = dat.StatsTemp[whichstat] + value endif set dat.PartStats[whichstat] = dat.PartStats[whichstat] + value call BJDebugMsg(R2S(dat.PartStats[whichstat])) if dat.PartStats[whichstat] >= 1.00 then if whichstat == 0 then call SetHeroStr(u,GetHeroStr(u,false)+1,true) elseif whichstat == 1 then call SetHeroAgi(u,GetHeroAgi(u,false)+1,true) elseif whichstat == 2 then call SetHeroInt(u,GetHeroInt(u,false)+1,true) endif set dat.PartStats[whichstat] = dat.PartStats[whichstat] - 1 endif endfunction private function Conditions takes nothing returns boolean return IsUnitType(GetTriggerUnit(),UNIT_TYPE_HERO) == true endfunction private function Actions takes nothing returns nothing local unit u = GetTriggerUnit() local integer i = H2I(u) local string s = I2S(i) local integer tmp_i = 0 local Data dat if GetStoredBoolean(gc,s+"BonusStats","Exist") then set dat = Data(GetStoredInteger(gc,s+"BonusStats","Struct")) if dat.StatsTemp[0] >= 1.00 then set tmp_i = R2I(dat.StatsTemp[0]) call SetHeroStr(u,GetHeroStr(u,false)-tmp_i,true) set dat.StatsTotal[0] = dat.StatsTotal[0] - tmp_i set dat.StatsTemp[0] = dat.StatsTemp[0] - tmp_i endif if dat.StatsTemp[1] >= 1.00 then set tmp_i = R2I(dat.StatsTemp[1]) call SetHeroAgi(u,GetHeroAgi(u,false)-tmp_i,true) set dat.StatsTotal[1] = dat.StatsTotal[1] - tmp_i set dat.StatsTemp[1] = dat.StatsTemp[1] - tmp_i
endif if dat.StatsTemp[2] >= 1.00 then set tmp_i = R2I(dat.StatsTemp[2]) call SetHeroInt(u,GetHeroInt(u,false)-tmp_i,true) set dat.StatsTotal[2] = dat.StatsTotal[2] - tmp_i set dat.StatsTemp[2] = dat.StatsTemp[2] - tmp_i endif set dat.PartStats[0] = 0. set dat.PartStats[1] = 0. set dat.PartStats[2] = 0. endif endfunction private function Init takes nothing returns nothing local integer i = 0 set tr = CreateTrigger() loop exitwhen i > 11 call TriggerRegisterPlayerUnitEvent(tr,Player(i),EVENT_PLAYER_UNIT_DEATH,null) set i = i + 1 endloop call TriggerAddCondition(tr,Condition(function Conditions)) call TriggerAddAction(tr,function Actions) endfunction endlibrary Just another Knockback library (*yawn*) Code library Knockback requires CheckLoc private keyword Data globals private timer CallbackTimer = CreateTimer() private Data array gdat private integer gi = 0 endglobals struct Data unit u real ix real iy real vx real vy real v real a real t real exit method onDestroy takes nothing returns nothing call PauseUnit(this.u,false) endmethod endstruct private function Callback takes nothing returns nothing local integer i = 0 local real x = .0 local real y = .0 local real dis = .0 local Data d loop exitwhen i >= gi set d = gdat[i] set d.t = d.t + .03 set dis = d.t * d.v + (.5 * d.a * (d.t*d.t)) set x = d.ix + dis * d.vx set y = d.iy + dis * d.vy if d.t > d.exit or not CheckLoc_Run(x,y) or GetWidgetLife(d.u) <= .405 then call d.destroy() set gi = gi - 1 set gdat[i] = gdat[gi] else call SetUnitX(d.u,x) call SetUnitY(d.u,y) set i = i + 1 endif endloop if gi == 0 then call PauseTimer(CallbackTimer) endif endfunction public function Create takes unit u, real angle, real v, real a returns nothing local Data d = Data.create() call PauseUnit(u,true) set d.u = u set d.ix = GetUnitX(u) set d.iy = GetUnitY(u) set d.vx = Cos(angle) set d.vy = Sin(angle) set d.v = v set d.a = a set d.t = 0 set d.exit = v/(a*-1) set gdat[gi] = d set gi = gi + 1 if gi == 1 then call TimerStart(CallbackTimer,0.03,true,function Callback) endif endfunction endlibrary
|
|
| |
Fireeye | Date: Thursday, 2008-10-30, 2:34 PM | Message # 2 |
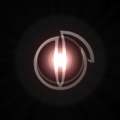 Private
Group: Moderators
Messages: 16
Status: Offline
| Let's see what i got else ..., ahh my Item List Library for Shops (NOT TESTED ONLINE YET!) Code library ItemList globals private constant integer ITEMS_PER_PAGE = 11 endglobals
struct Items integer array ItemID [ITEMS_PER_PAGE] integer array InitialCharges [ITEMS_PER_PAGE] integer array MaxCharges [ITEMS_PER_PAGE] integer currentslot = 0 method CheckForItem takes integer itemid returns boolean local integer i = 0 loop exitwhen i >= .currentslot if .ItemID[i] == itemid then return true endif set i = i + 1 endloop return false endmethod method AddItemTypeToList takes integer itemid, integer initamount, integer maxamount returns nothing if not .CheckForItem(itemid) then set .ItemID[.currentslot] = itemid set .InitialCharges[.currentslot] = initamount set .MaxCharges[.currentslot] = maxamount set .currentslot = .currentslot + 1 else call BJDebugMsg("TRYING TO ADD AN ITEM TWICE TO AN ITEM LIST!") endif endmethod method GetIndex takes integer itemid returns integer local integer i = 0 loop exitwhen i >= .currentslot if .ItemID[i] == itemid then return i endif set i = i + 1 endloop return -1 endmethod method RemoveItemFromList takes integer itemid, boolean Check returns nothing local integer i = 0 if .currentslot > 0 then if Check then if not .CheckForItem(itemid) then call BJDebugMsg("TRYING TO REMOVE AN NON-EXISTING ITEM FROM AN ITEM LIST!") return endif endif set i = .GetIndex(itemid) set .currentslot = .currentslot - 1 set .ItemID[i] = .ItemID[.currentslot] set .MaxCharges[i] = .MaxCharges[.currentslot] set .InitialCharges[i] = .InitialCharges[.currentslot] else call BJDebugMsg("TRYING TO REMOVE AN ITEM FROM AN EMPTY ITEM LIST!") return endif endmethod method AddListToShop takes unit u returns nothing local integer i = 0 loop exitwhen i >= .currentslot call AddItemToStock(u,.ItemID[i],.InitialCharges[i],.MaxCharges[i]) set i = i + 1 endloop endmethod method AddListToShopForPlayer takes unit u, player p returns nothing local integer i = 0 loop exitwhen i >= .currentslot if GetLocalPlayer() == p then call AddItemToStock(u,.ItemID[i],.InitialCharges[i],.MaxCharges[i]) endif set i = i + 1 endloop endmethod method RemoveListFromShop takes unit u returns nothing local integer i = 0 loop exitwhen i >= .currentslot call RemoveItemFromStock(u,.ItemID[i]) set i = i + 1 endloop endmethod method RemoveListFromShopForPlayer takes unit u, player p returns nothing local integer i = 0 loop exitwhen i >= .currentslot if GetLocalPlayer() == p then call RemoveItemFromStock(u,.ItemID[i]) endif set i = i + 1 endloop endmethod endstruct endlibrary There are several other systems, but they are embed into the triggers most time, i'll look if i can extract some of em.
|
|
| |
Fireeye | Date: Monday, 2008-11-03, 2:57 PM | Message # 3 |
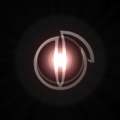 Private
Group: Moderators
Messages: 16
Status: Offline
| Darn, just found an error in my checkloc library, update will be coming today. And here are some description: - GameCache Do i really need to explain this? It's just some library with game cache in it and the H2I function. I personally dislike the gamecache, however there are situations where it is acceptable, due it can be faster than a loop through. - Check Loc Just checks if the location @ Loc(x,y) is pathable or not. (forgot to change the item movement to array instead of x,y , will be changed soon) - LightningFade This one changes to color of your lightning from the initial, or any color you choose to the target color of n seconds. - BonusStats Not much to say, you just call BonusStats_Add() to add an attribute bonus to a unit, you can declare which attribute and if it should be reset on death. The attributes are Strenght (0), Agility (1) and Intelligence (2). You only need to call the Add function due the reset will automatically fire. - ItemList This one is the tricky due i didn't really test it online (it may cause desync / server split). It basically allows you to add item raw codes and its charges to a struct array, which then can be used to allow a shop to sell different items to different players. You call Items.create() first (don't forget to assign it to a variable) and afterwards, you call variable.AddItemTypeToList() to add up to n items to the list, when you're done adding, you use the variable.AddListToShop() or the variable.AddListToShopForPlayer() command, you can also remove items from the item list or remove an item list from a shop again.
|
|
| |
Hanky | Date: Tuesday, 2008-11-04, 1:49 PM | Message # 4 |
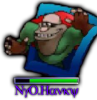 Lieutenant colonel
Group: Moderators
Messages: 116
Status: Offline
| Seems to be interesting I currently just found one thing: Code private function Conditions takes nothing returns boolean return IsUnitType(GetTriggerUnit(),UNIT_TYPE_HERO) == true endfunction make it to: Code private function Conditions takes nothing returns boolean return IsUnitType(GetTriggerUnit(),UNIT_TYPE_HERO) endfunction But else it could be useful. For example the knockback ;).
Made by Smoe reworked by GiR aka Darkt3mpl3r
|
|
| |
Fireeye | Date: Tuesday, 2008-11-04, 9:58 PM | Message # 5 |
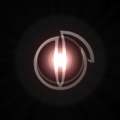 Private
Group: Moderators
Messages: 16
Status: Offline
| IsUnitType can bug in some cases (i think it was a building comparision) therefore i prefer the == true to be on the safe side. Can look for the actual wc3c thread. CheckLoc will be up to date in a few minuts. ---Edit--- just found another error in the Knockback function, but i fixed it already and updated the first post.
|
|
| |
Hanky | Date: Wednesday, 2008-11-05, 10:09 AM | Message # 6 |
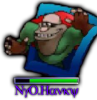 Lieutenant colonel
Group: Moderators
Messages: 116
Status: Offline
| Ah yes I forgot about that, I read that somewhere too. But whatever good job so far ;).
Made by Smoe reworked by GiR aka Darkt3mpl3r
|
|
| |
Fireeye | Date: Wednesday, 2008-11-05, 3:39 PM | Message # 7 |
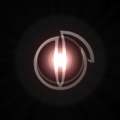 Private
Group: Moderators
Messages: 16
Status: Offline
| I'm currently working on an updated version of the knockback which allows a positive acceleration. e.g. you want a unit to charge a point. It's function syntax will be propably Knockback_Create(<unit>,<angle>,<initial speed>,<acceleration>,<target speed>) i think i gonna use the entire calculations already used, but instead of v/(a*-1) i gonna change it to (v0-v1)/(a*-1) which should work for positive acceleration too then. I'll post when up to date. BTW Anyone got any hero ideas? I'm almost done with the necromancer posted by Darkt3mpl3r (but a bit changed).
|
|
| |
Hanky | Date: Thursday, 2008-11-06, 1:07 AM | Message # 8 |
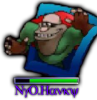 Lieutenant colonel
Group: Moderators
Messages: 116
Status: Offline
| Hmm I never got full ideas of hero themes, I just got single spell ideas. But hmm... tomorrow I got school and next day again school I think there I will have some time to think about some hero theme ideas.
Made by Smoe reworked by GiR aka Darkt3mpl3r
|
|
| |
|